CSS Tutorial
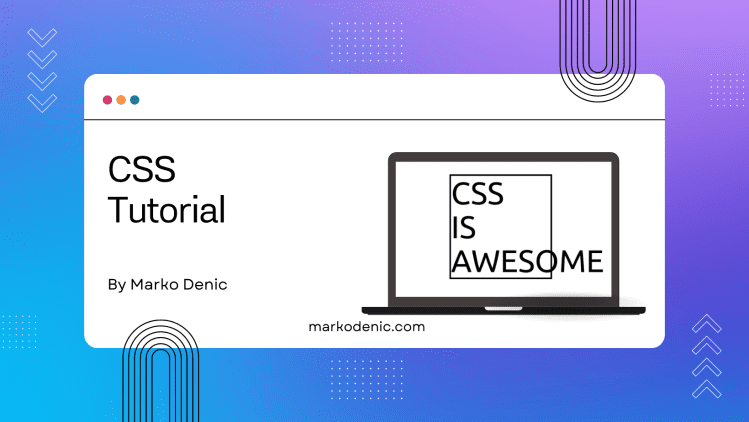
Table of contents
1. Introduction
2. Basics of CSS
3. CSS Properties and Values
4. CSS Selectors
5. CSS Box Model
6. CSS Layout
7. CSS Media Queries
8. CSS Best Practices
9. CSS Preprocessors
10. Conclusion
1. Introduction
Definition of CSS (Cascading Style Sheets)
CSS, or Cascading Style Sheets, is a styling language used in web development to control the appearance and layout of web pages. It defines how HTML elements should be displayed, including aspects like colors, fonts, spacing, and positioning.
Importance of CSS in web development
CSS plays a crucial role in web development by separating the presentation layer from the content layer. It allows developers to create visually appealing and consistent designs across multiple web pages. CSS also improves website accessibility, search engine optimization, and overall user experience.
Purpose of the article
The purpose of this article is to provide an accessible introduction to CSS for beginners in web development. It aims to explain the core concepts of CSS, its syntax, selectors, properties, and values. By the end of the article, readers will have a solid understanding of CSS and be equipped to apply basic styling to their web pages.
2. Basics of CSS
Separation of style and structure
CSS separates the style and structure of a web page, allowing developers to modify the appearance of elements without altering the underlying HTML structure. This separation enhances code maintainability and provides flexibility for design changes.
CSS syntax and selectors
CSS syntax consists of selectors, which target specific elements, and declarations, which define the styles applied to those elements. Selectors can target elements based on their tag names, classes, IDs, attributes, or hierarchical relationships.
Inline styles vs. internal stylesheets vs. external stylesheets
CSS can be applied in different ways. Inline styles are directly embedded within HTML tags using the style
attribute. Internal stylesheets are placed within the <style>
tags in the HTML file. External stylesheets are separate CSS files linked to the HTML file using the <link>
tag.
Understanding the box model
The box model is a fundamental concept in CSS that defines how elements are rendered and how their dimensions are calculated. It consists of content, padding, border, and margin. Understanding the box model is essential for proper element positioning and spacing.
3. CSS Properties and Values
Text-related properties
CSS provides properties to control text appearance, including font properties, color properties, text alignment, text decoration, and text transformations.
Example:
p {
font-family: Arial, sans-serif;
color: #333;
font-size: 16px;
text-align: center;
text-decoration: underline;
}
Box properties
Box properties control the dimensions, spacing, and border of elements. These properties include width, height, margin, padding, border, and box-sizing.
Example:
div {
margin: 10px;
padding: 20px;
border: 1px solid #000;
width: 200px;
height: 150px;
}
Background properties CSS offers various background properties to define the background color, image, repeat behavior, positioning, and size of elements.
Example:
body {
background-color: #f2f2f2;
background-image: url("background.jpg");
background-repeat: no-repeat;
background-position: center;
}
Positioning properties
CSS provides positioning properties like position, top, bottom, left, and right, which allow elements to be positioned relative to their containing elements or the viewport.
Display properties
Display properties determine how elements are rendered. Common display values include block, inline, inline-block, flex, and grid. These properties affect the flow and positioning of elements on the page.
CSS units
CSS supports different units for specifying measurements, such as pixels (px), percentages (%), em, rem, and viewport-relative units like vw and vh.
4. CSS Selectors
Element selectors
Element selectors target specific HTML elements based on their tag names. For example, h1
selects all <h1>
headings on the page.
Class selectors
Class selectors target elements based on the class attribute assigned to them. They are denoted by a dot followed by the class name. For example, .btn
selects all elements with the class “btn”.
ID selectors
ID selectors target elements with a specific ID attribute. They are denoted by a hash symbol followed by the ID name. For example, #logo
selects the element with the ID “logo”.
Attribute selectors
Attribute selectors target elements based on specific attribute values.
Example:
input[type="text"] {
border: 1px solid #999;
}
Pseudo-classes and pseudo-elements Pseudo-classes select elements based on their state or position, while pseudo-elements style specific parts of an element. Common examples include :hover
, :active
, :first-child
, and ::before
.
5. CSS Box Model
Understanding the box model concept
The box model is a fundamental concept in CSS that defines how elements are rendered and how their dimensions are calculated. It consists of content, padding, border, and margin. Understanding the box model is essential for proper element positioning and spacing.
Box model properties
Box model properties allow developers to control the dimensions and spacing of elements. These properties include width
, height
, margin
, padding
, and border
.
Box sizing
Box sizing determines how the dimensions of an element are calculated. The two common values are content-box
, which includes only the content area, and border-box
, which includes the content, padding, and border within the specified dimensions.
Example:
.box {
box-sizing: border-box;
width: 200px;
height: 150px;
padding: 10px;
border: 1px solid #000;
margin: 20px;
}
6. CSS Layout
Floats and clearing floats
Floats allow elements to be positioned to the left or right, enabling text and other elements to flow around them. Clearing floats is necessary to ensure that subsequent elements are not affected by the floated elements.
Flexbox layout
Flexbox is a powerful layout system that provides a flexible way to distribute space among elements. It simplifies the alignment, ordering, and resizing of elements within a container.
Example:
.container {
display: flex;
justify-content: center;
align-items: center;
}
Grid layout
CSS Grid is a two-dimensional layout system that allows for precise control over the positioning and alignment of elements in a grid-like structure. It provides powerful capabilities for creating complex layouts.
Responsive design using media queries
Media queries enable the creation of responsive designs that adapt to different screen sizes and devices. By defining specific CSS rules based on screen widths, developers can customize the layout and styles accordingly.
7. CSS Media Queries
Definition and purpose of media queries
Media queries allow developers to apply specific CSS rules based on the characteristics of the user’s device or viewport, such as screen width, height, and orientation. They are essential for creating responsive and mobile-friendly designs.
Writing media queries
Media queries are written using the @media
rule followed by the desired conditions and CSS rules inside the block. Common conditions include max-width
, min-width
, and orientation
.
Example:
@media (max-width: 768px) {
/* CSS rules for screens up to 768px */
}
Examples of responsive design using media queries
Media queries can be used to create responsive layouts, hide or show specific elements, adjust font sizes, and modify other styles to optimize the user experience across different devices and screen sizes.
8. CSS Best Practices
Using CSS reset or normalize
CSS reset or normalize stylesheets are used to reset or normalize the default styles applied by different browsers, ensuring consistent rendering across platforms.
Organizing and structuring CSS code
Organizing CSS code improves readability and maintainability. Common approaches include using meaningful class names, grouping related styles, and organizing stylesheets into separate files.
Using shorthand properties
Shorthand properties allow developers to set multiple related properties using a single line of code. They help reduce redundancy and improve code efficiency.
Avoiding global styles
Minimize the use of global styles that affect all elements on the page. Instead, use more specific selectors to target individual elements or groups of elements. This helps avoid unintended style conflicts and makes code easier to manage and maintain.
Efficiently targeting elements with selectors
Select efficient selectors that target elements directly or use classes and IDs for more specific targeting. Avoid using overly complex or inefficient selectors that can slow down rendering.
9. CSS Preprocessors (optional)
Introduction to CSS preprocessor
CSS preprocessors like Sass (Syntactically Awesome Style Sheets) or Less are extensions of CSS that provide additional features and functionality. They introduce variables, nesting, mixins, and more, making CSS code more modular and easier to maintain.
Benefits and features of using preprocessors
Preprocessors offer advantages such as code reusability, improved organization, and enhanced readability. They allow for the use of functions, conditional statements, and loops, enabling more dynamic and powerful CSS.
Working with variables, mixins, and nesting
Variables in preprocessors enable the definition and reuse of values throughout the stylesheet. Mixins are reusable blocks of CSS code that can be included in multiple styles. Nesting allows for nesting selectors within each other, reducing repetition and improving readability.
10. Conclusion
Recap of key concepts covered in the article
This article provided an introduction to CSS, covering essential topics such as syntax, selectors, properties, the box model, layout techniques, media queries, and best practices. It emphasized the importance of CSS in web development and explained how CSS separates style from structure.
Encouragement to continue exploring CSS and web development
CSS is a powerful tool for creating visually appealing and responsive websites. By continuing to learn and explore CSS, developers can enhance their skills and unlock endless possibilities in web design.